A Basic Avatar
The Brief.
For this task I was required to implement a basic playable avatar for a 3D platformer. The avatar must be able to stand, walk, run, jump and attack, any more functionality will be deemed as additional behaviour. The character model and animations that I chose to use were from the RPG Character Mecanim Animation Pack FREE which can be installed from the Unity Asset Store. This pack includes a very basic character model and a wide range of animations for it, my task was to use create a smooth feeling character controller using C# and utilize Unity’s animator system to create convincing transitions between each animation.
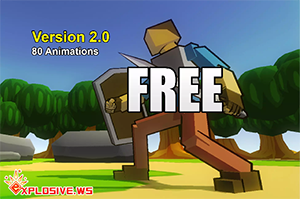
Implementing simple character movement.
To begin with I added essential components to the player object, a ‘rigidbody’ so it is effected by physics and a collider to ensure the player doesn’t walk or fall through meshes. I created some methods in a player controller script to get input from the user and move the player accordingly. I chose to design my project for the use of a PlayStation 4 controller, this meant I needed to change some of the parameters within Unity’s input settings. Movement along the X and Z axes are by default called the horizontal and vertical axes respectively, each of these input axes can take a value from -1 to 1 depending on the position of the left analog stick on the controller. Using these values I could determine how fast the player moves, as I could multiply the velocity of the player with the value produced from each of the input axes. To animate the player I could get the magnitude of his velocity and use this to determine whether the walk or run animation should be played. For example if the magnitude of his velocity was greater than five I could transition from walking to running and vice versa. The rotation of the player is also set to the direction of hid velocity in both the X and Z axes.
Dealing with jumping.
Getting the character to jump was as simple as detecting a button press from the user and then applying a force to the players velocity on the Y axis. To prevent the player from repeatedly jumping in mid air, I needed some way to check whether he was actually on the ground or not before jumping. To do this I used the ‘IsGrounded()’ method below. This method checks for any colliders with the ‘Walkable Layer’ within a specified radius, if there is it will return true and if not it will return false. It made most sense for this radius to be located at the players feet. The image to the right shows the area of which detects the ground using the red sphere.
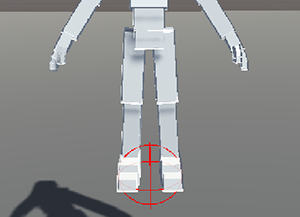
The Jump method itself has a little more functionality than I initially mentioned. As can be seen in the code below it checks if the player has any extra jumps available before allowing them to jump again. This is because in later stages of this project I am expected to create collectables for the player to collect within the world. One of these collectables should give the player the ability to double jump, so I decided to implement the functionality of this double jump feature now rather than later. Although the player is only expected to double jump, the beauty of my method is that the ‘extraJumpCount’ integer can be set to any number, so if this project was expanded upon the player could triple jump or even more.
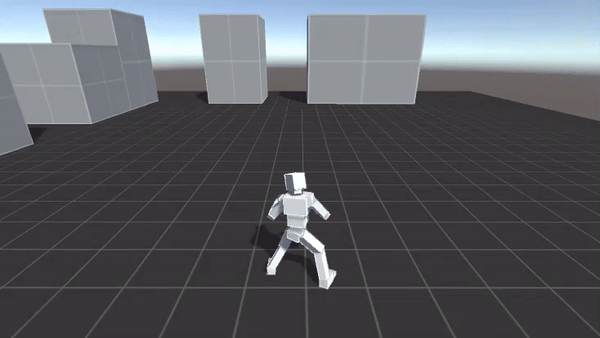
Some issues I encountered and how I overcame them.
Initially I dealt with all physics and input within the update function, but this lead to my character’s jumping being very inconsistent as each jump would differ in height. After reading about the issue I came to realise that all physics related calculations should be within ‘FixedUpdate’ to keep the height of character’s jump consistent, along with all other physics within my game. After moving a large chunk of my code into the ‘LateUpdate’ function to solve this, I encountered another issue. This was that my input wasn’t always being recognised correctly, after more research I discovered that checking for input should be executed within the ‘Update’ function where it initially was before I moved it into the ‘LateUpdate’. This meant I had to separate the physics and input calculations into different functions, and I solved this by simply creating a boolean value that that was only true when the user presses the button to jump.
Adding extra functionality and animations.
Although I have a character that is able to stand, walk, run and jump there is more functionality I want to include to create a more advanced system. I will explain how I implemented the extra features along with images and animations demonstrating them.
Forward roll.
The forward roll is an ability I plan to use as a collectable once I have a functioning collectable system. Although I thought now would be a good time to work on the functionality and animation of the forward roll as I’m working on the player controller. I used Unity’s animation state machine behaviour system to add extra functionality to the player during the roll animation, this included adding a force to the player in the direction of the roll and decreasing the size of the player’s collider to fit the character model. The function below is called each time the roll animation starts
The double jump and landing animation.
To add a higher quality feel to the character controller I chose to add a flip animation each time the player double jumps. This animation triggers automatically as long as the user jumps more than once. I also included an extra animation each time the player lands from a jump, this makes each landing feel lot more impactful on the player. The way in which this animation is triggered is very similar method used to check if the player is grounded or not. If the player is a small distance from the ground and his Y velocity is less than zero, than the animation will be played. The transition from each animation can be seen below in the image of the player’s animator controller.