Collectables with Particles
The Brief.
For this task I was required to implement a couple collectables that will give the player power ups once obtained, one that should provide a speed boost and the other a double jump ability. Both of these should appear visually magical and communicate with the user the result of the power up. Both power ups should provide their unique benefit for a certain amount of time during the gameplay before they eventually wear off. To test the result of these power ups I will need to expand upon my initial environment so I can gauge the advantage that the player is given and how that effects the gameplay. Some things that I will need to take note of during this task will be:
- Collectables often have a lot in common. How can I make them visually different given that the designer will probably need many more?
- How should the power ups behave when more than one is active?
- How should the power up behave when the player collects one after the other within a short time period?
A screen capture of Jack and Daxter gameplay showing the visuals of a collectable and the power up effect.
My collectable system.
Firstly I made a parent class Collectable, this class would contain all the data and functionality that is shared between all collectables within my game. This class detects collision with the player, the duration of the power up’s effect, a method to deactivate the collectable and another to reset the power up’s effect. The snippet of code below is the timer functionality. The method initially checks if the player has collected the power up and if they have then the timer will be begin incrementing with time, once the timer exceeds powerUpDuration variable then the power up effect is removed using the ResetPowerUp function call. The boolean checking if the player has the power up is then set to false, leading to the timer value being reset back to zero.
Each collectable object that I create within my game will have a separate script attached that will inherit from the Collectable class. Each of these child classes will include the functionality of the effect specified to that collectable, along with removing the collectable’s effect once the timer runs out. I achieved this by the use of override functions that are called within the parent Collectable class, each of these functions are specific to collectable object they are attached to. The code below shows the DoubleJump class, with a method that is called once the double jump collectable is obtained, along with another to remove the effects of the double jump. The logic of the double jump itself can be seen in my previous blog post, ‘A Basic Avatar’.
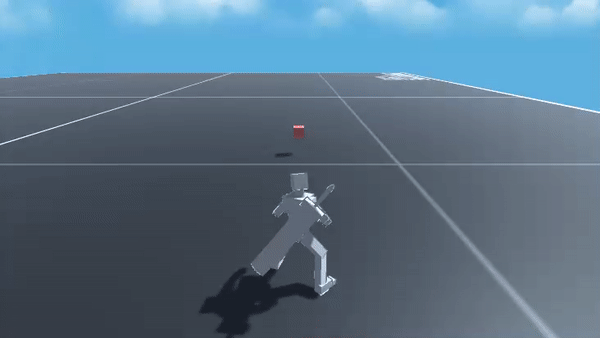
Creating more collectables.
To meet the criteria given in the brief I was required to make at least one more collectable which provide the speed boost ability. With the collectable system place it was simple for me to create and add any more collectables to my world, all that was required for me to do was to make a SpeedBoost class which has a near identical structure to the DoubleJump class. The only difference being the logic of the power up’s effect as rather than giving the player the ability to double jump, a speed multiplier variable is used to increase the movement speed of the player.
To expand and add additional behaviour to my game I decided to add a third and final collectable. This collectable grants the player with the ability to forward roll, it may seem underwhelming in comparison to the other two powers but the forward roll allows the player to move faster and decrease they’re surface area meaning they can fit through narrow openings. The logic that differentiates this collectable from the previous two is that a roll animation is played, I utilised a State Machine Behaviour script to add additional functionality whilst this animation was being played. The logic for the forward roll functionality can be seen in my previous blog post, ‘A Basic Avatar’.
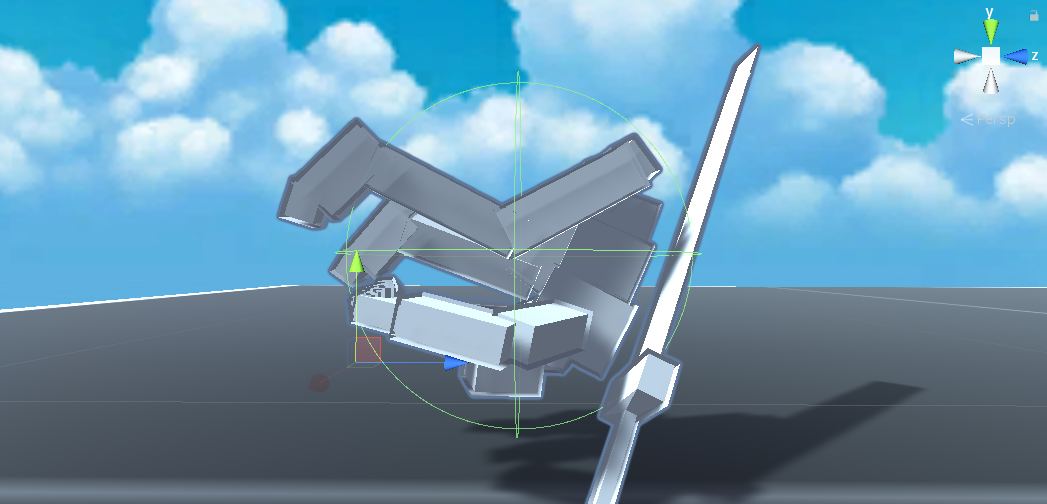
An image showing the character’s collider decreasing in size during the forward roll.
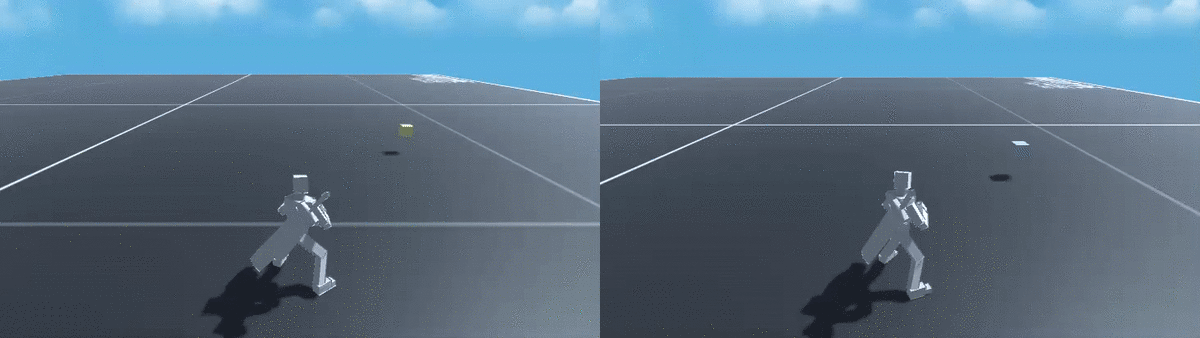
A GIF showing the speed boost effect (left) and forward roll effect (right) side by side.
Designing the collectables and particle systems.
As part of the brief I was required to make each collectable distinguishable from one another, I did this by using different particle systems and colours for each of them.
Collectable | Colour | Particle System |
---|---|---|
Double Jump | Red | Fire / Explosion |
Speed Boost | Yellow | Lightning Pulse / Trail |
Forward Roll | Blue | Water Fountain / Splash |
A table showing the colour and particle system of each collectable.
To create each of the particle systems I followed the following tutorials:
- Fire Particle System (Unity VFX Tutorials - 08 - Basics (Fire) by Sirhaian’Arts )
- Lightning Particle System (Game VFX tutorial - Electricity effects - Unity3d by Hovl Studio)
- Water Particle System (Unity 3d Tutorials - Particle FX - #2 Water Fountain by ChromeFXFilms)
I made some minor adjustments to each of the systems such as changing colours and parameters to make them more suited to my needs. The result of each of the collectable items can be seen below.
As can be seen in the GIF I also added some movement and rotation values to each of the collectables to give them a little more life, I used the following code to achieve this effect.
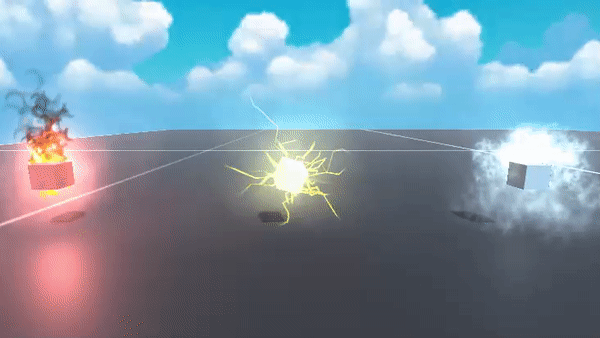
Check out my progress so far.
As can be seen in the video, I have changed my environment to test my current power up abilities. There is also another particle system that is played during the speed boost power up, this is the warp effect around the camera that creates the effect that the player is moving faster. I followed this tutorial by Mirza to create the effect.